How to Build Android Mobile Apps. The world of mobile applications is booming. Android apps are integral to our daily lives, from productivity tools and entertainment platforms to essential services and communication hubs. Ever wondered how these apps are made? Building an Android app might seem daunting, but anyone can bring their app idea to life with the right tools, knowledge, and dedication.
This comprehensive guide will walk you through the entire process, from initial concept to launching your app on the Google Play Store. Whether you’re a complete beginner or have some programming experience, this post will equip you with the understanding and resources to embark on your Android app development journey.
How to Build Android Mobile Apps
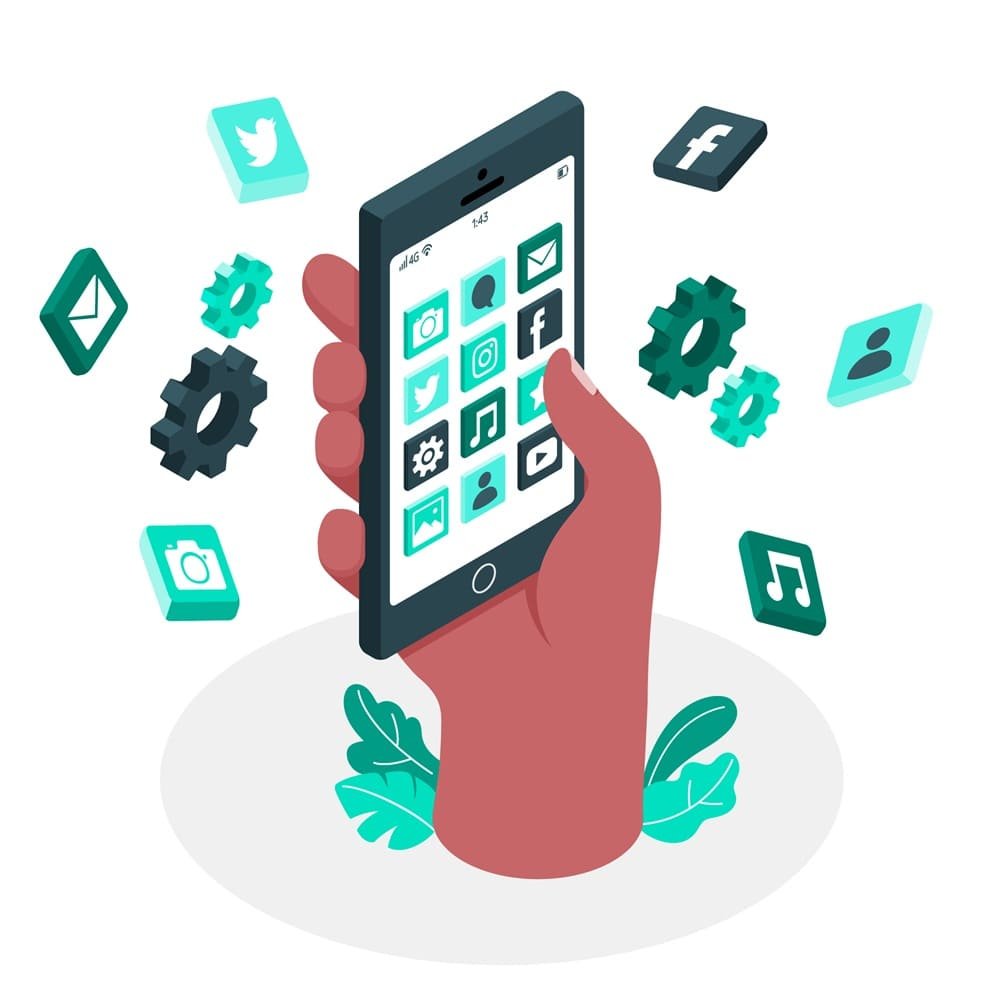
I. Laying the Foundation: Preparation and Planning
Before diving into code, it’s crucial to lay a solid foundation. This involves careful planning and understanding the core concepts involved.
- 1. Define Your App Idea:
- The Problem/Solution: Start by clearly defining the problem your app solves or the need it fulfills. What gap are you filling in the market?
- Target Audience: Who will use your app? Understanding your target demographic will influence design choices, features, and marketing strategies.
- Unique Selling Proposition (USP): What makes your app stand out from the competition? Identify a unique feature or aspect that will attract users.
- Monetization Strategy (Optional): How will your app generate revenue? Consider options like in-app advertisements, freemium models, subscriptions, or one-time purchases.
- 2. Market Research:
- Competitor Analysis: Research existing apps in your niche. Identify their strengths and weaknesses to find opportunities for improvement or differentiation.
- Keyword Research: Identify relevant keywords that users might use when searching for apps like yours on the Google Play Store. This will be crucial for App Store Optimization (ASO).
- User Reviews: Analyze user reviews of competing apps to understand their pain points and unmet needs. This can provide valuable insights for feature development.
- 3. Wireframing and Prototyping:
- Wireframing: Create low-fidelity sketches or digital mockups of your app’s screens. Focus on the layout, content, and user flow. Tools like Balsamiq, Figma, and Adobe XD can be helpful.
- Prototyping: Develop an interactive prototype that simulates the user experience. This allows you to test the usability of your app and identify potential issues early on. Tools like InVision and Marvel are popular choices.
- User Feedback: Gather feedback on your wireframes and prototypes from potential users. This will help you refine your design and ensure it meets their needs.
- 4. Choose Your Development Approach:
- Native Development: Building apps specifically for the Android platform using Java or Kotlin. Offers the best performance and access to all device features but can be more complex and require platform-specific knowledge.
- Cross-Platform Development: Using frameworks like React Native, Flutter, or Xamarin to write code once and deploy it on both Android and iOS. Can be faster and more cost-effective, but may have limitations in performance or access to native features.
- Hybrid Development: Combining web technologies (HTML, CSS, JavaScript) with native wrappers to create apps. Offers quick development but can suffer from performance issues.
II. Choosing Your Development Environment and Tools
Once you have a plan, you’ll need to set up your development environment. Here are the essentials:
- 1. Android Studio:
- The Official IDE: Android Studio is the official Integrated Development Environment (IDE) for Android development. It provides all the necessary tools and features for coding, debugging, testing, and building your app.
- Download and Installation: Download Android Studio from the official Android Developers website (https://developer.android.com/studio). Follow the installation instructions specific to your operating system (Windows, macOS, or Linux).
- SDK Manager: Android Studio includes an SDK (Software Development Kit) Manager that allows you to download and manage different versions of the Android operating system. This is crucial for testing your app on various Android devices.
- Emulator: Android Studio comes with a built-in emulator that allows you to test your app on a virtual Android device. This eliminates the need to constantly deploy your app to a physical device during development.
- 2. Programming Languages:
- Java: Java was the primary language for Android development for many years. It’s a robust and mature language with a large community and extensive libraries. While still supported, Kotlin is now the preferred language.
- Kotlin: Kotlin is a modern, concise, and interoperable language that is officially supported by Google for Android development. It offers several advantages over Java, including null safety, extension functions, and coroutines. Learning Kotlin is highly recommended for new Android developers.
- XML: XML (Extensible Markup Language) is used to define the layout and user interface of your Android app. You’ll use XML to create the structure and appearance of your app’s screens, including buttons, text views, and images.
- 3. Other Useful Tools:
- Git: A version control system that allows you to track changes to your code and collaborate with other developers.
- Gradle: A build automation tool that automates the process of compiling, packaging, and deploying your Android app.
- Firebase: A comprehensive platform for building and managing mobile apps. Offers features like authentication, real-time database, cloud storage, and analytics.
- Postman/Insomnia: Tools for testing APIs (Application Programming Interfaces) if your app interacts with a backend server.
III. Building Your Android App: The Core Steps
Now that you have your environment set up, it’s time to start building your app.
- 1. Creating a New Project:
- Project Setup: In Android Studio, create a new project and choose a suitable template (e.g., Empty Activity, Basic Activity).
- Package Name: Define a unique package name for your app. This is usually in the reverse domain name format (e.g., com.example.myapp).
- Minimum SDK: Choose the minimum Android SDK version your app will support. This determines the range of devices your app can run on.
- Language Selection: Select either Java or Kotlin as your programming language.
- 2. Designing the User Interface (UI):
- XML Layout Files: Use XML layout files to define the structure and appearance of your app’s screens.
- UI Components: Add UI components like TextViews, Buttons, EditTexts, ImageViews, and RecyclerViews to your layouts.
- Layout Managers: Use layout managers like LinearLayout, RelativeLayout, ConstraintLayout, and FrameLayout to arrange the UI components within your layouts.
- Styling and Themes: Apply styles and themes to customize the appearance of your UI components and ensure a consistent look and feel.
- Responsive Design: Design your UI to adapt to different screen sizes and orientations. Use responsive layout techniques and density-independent units (dp) to ensure your app looks good on all devices.
- 3. Implementing Functionality:
- Activities: Activities represent individual screens or parts of your app’s user interface.
- Fragments: Fragments are reusable UI components that can be embedded within Activities.
- Intents: Intents are used to communicate between Activities and other components of your app. They can be used to start new Activities, send data, and request actions.
- Services: Services run in the background and perform long-running operations without user interaction.
- Broadcast Receivers: Broadcast Receivers listen for system-wide events and perform actions in response.
- Data Persistence: Implement data persistence using options like:
- Shared Preferences: For storing small amounts of key-value data.
- SQLite Database: For storing structured data in a local database.
- Room Persistence Library: A more modern and recommended approach for working with SQLite databases.
- Cloud Storage (Firebase): For storing data in the cloud.
- 4. Connecting to APIs (If Applicable):
- REST APIs: Use REST APIs to fetch data from external servers or services.
- Retrofit: A popular library for making network requests to REST APIs.
- JSON Parsing: Parse JSON data returned by REST APIs using libraries like Gson or Moshi.
- Asynchronous Operations: Perform network requests asynchronously to avoid blocking the main thread and ensure a smooth user experience.
- 5. Handling User Input:
- Event Listeners: Use event listeners to respond to user actions like button clicks, text changes, and gestures.
- Input Validation: Validate user input to prevent errors and ensure data integrity.
- 6. Testing and Debugging:
- Unit Tests: Write unit tests to verify the correctness of individual components and functions.
- UI Tests: Write UI tests to verify the behavior of your app’s user interface.
- Debugging: Use the Android Studio debugger to identify and fix bugs in your code.
- Logcat: Use Logcat to view system logs and track down errors.
- Emulator Testing: Thoroughly test your app on the Android emulator with different configurations and screen sizes.
- Real Device Testing: Test your app on a variety of real Android devices to ensure compatibility and performance.
IV. Polishing and Optimization
Once your app is functional, it’s important to polish and optimize it for a better user experience.
- 1. Performance Optimization:
- Reduce Memory Usage: Optimize your code to minimize memory usage and prevent memory leaks.
- Optimize Network Requests: Minimize the number and size of network requests to improve performance.
- Use Caching: Cache data to reduce the need for repeated network requests.
- Avoid Blocking the Main Thread: Perform long-running operations in background threads to avoid blocking the main thread and ensure a smooth user experience.
- 2. User Interface (UI) Enhancements:
- Animations and Transitions: Add animations and transitions to make your app more visually appealing and engaging.
- Material Design: Follow Material Design guidelines to create a consistent and intuitive user interface.
- Accessibility: Make your app accessible to users with disabilities by providing alternative text for images, using appropriate color contrast, and supporting screen readers.
- 3. Localization:
- String Resources: Use string resources to store all text in your app.
- Localization Support: Add localization support to make your app available in multiple languages.
- 4. Battery Optimization:
- Minimize Background Activity: Minimize background activity to reduce battery drain.
- Use Battery-Aware APIs: Use battery-aware APIs to optimize your app’s behavior based on the device’s battery level.
V. Publishing Your App on the Google Play Store
After rigorous testing and optimization, you’re ready to share your creation with the world!
- 1. Create a Google Play Developer Account:
- Registration: Register for a Google Play Developer account on the Google Play Console (https://play.google.com/console).
- Payment: Pay the one-time registration fee.
- 2. Prepare Your App Metadata:
- App Title: Choose a descriptive and keyword-rich app title.
- Short Description: Write a concise and compelling short description that highlights the key features of your app.
- Long Description: Write a detailed and informative long description that provides more information about your app and its benefits.
- Screenshots and Videos: Upload high-quality screenshots and videos that showcase your app’s features and user interface.
- Icon: Create a visually appealing and recognizable app icon.
- Category and Tags: Choose the appropriate category and tags for your app.
- Content Rating: Determine the appropriate content rating for your app based on its content.
- Privacy Policy: Provide a link to your app’s privacy policy.
- 3. Build and Upload Your App Bundle:
- Generate Signed APK/AAB: Generate a signed APK (Android Package Kit) or AAB (Android App Bundle) of your app. AAB is the recommended format as it allows Google Play to optimize the app for each user’s device, reducing download sizes.
- Upload to Google Play Console: Upload the APK/AAB to the Google Play Console.
- 4. Set Pricing and Distribution:
- Pricing: Choose a pricing model for your app (free or paid).
- Distribution: Choose the countries where you want to distribute your app.
- Staged Rollout: Consider using a staged rollout to gradually release your app to a small percentage of users before releasing it to everyone.
- 5. Review and Publish:
- Google Play Review: Google Play will review your app to ensure it meets their policies and guidelines.
- Publication: Once your app is approved, you can publish it to the Google Play Store.
VI. Post-Launch: Maintaining and Improving Your App
Launching your app is just the beginning. Ongoing maintenance and improvement are crucial for long-term success.
- 1. Monitor App Performance:
- Google Play Console Analytics: Use the Google Play Console analytics to track your app’s performance, including downloads, active users, retention rate, and crash reports.
- Crashlytics/Firebase Crash Reporting: Use crash reporting tools to identify and fix crashes in your app.
- Performance Monitoring Tools: Use performance monitoring tools to identify performance bottlenecks and optimize your app’s performance.
- 2. Gather User Feedback:
- Google Play Store Reviews: Monitor user reviews on the Google Play Store and respond to feedback.
- In-App Feedback Mechanisms: Implement in-app feedback mechanisms to allow users to easily provide feedback.
- User Surveys: Conduct user surveys to gather more in-depth feedback.
- 3. Update Your App Regularly:
- Bug Fixes: Fix bugs and address user feedback promptly.
- New Features: Add new features and improvements based on user feedback and market trends.
- Security Updates: Apply security updates to protect your app and users from vulnerabilities.
- Android SDK Updates: Update your app to the latest Android SDK version to take advantage of new features and improvements.
- 4. App Store Optimization (ASO):
- Keyword Optimization: Continuously optimize your app’s keywords to improve its visibility in the Google Play Store.
- App Title and Description Optimization: Optimize your app’s title and description to attract users.
- Screenshot and Video Optimization: Optimize your app’s screenshots and videos to showcase its features and user interface.
Conclusion: Your Journey to App Development Success
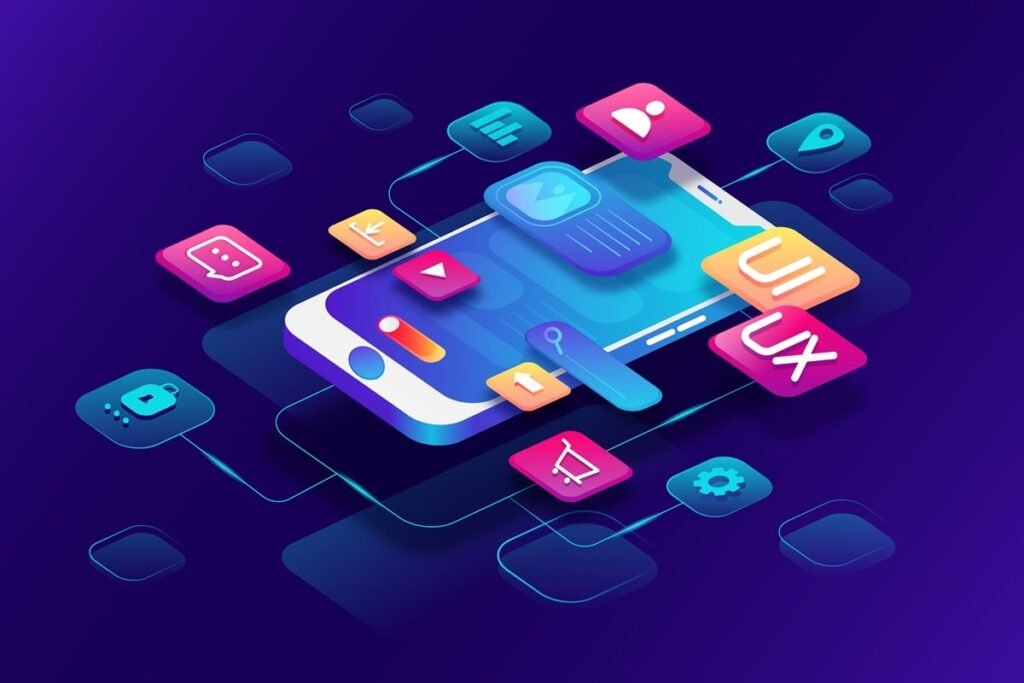
Building an Android app is a challenging but rewarding endeavor. By following the steps outlined in this guide, you can turn your app idea into a reality. Remember to embrace the learning process, stay persistent, and continuously improve your app based on user feedback and market trends. The world of app development is constantly evolving, so continue learning and exploring new technologies to stay ahead of the curve. Good luck, and happy coding!